A More Modern Approach
Now, it's very common to see websites using languages like JavaScript, a subset of Java, to make their websites interactive.
JavaScript can be used virtually anywhere - dragging and dropping elements on the screen, changing the size, validating forms... the list goes on.
What is most important is how this is structured. Tables, rigid and powerful as they are, are unsuitable for website design, especially so if there are any dynamic elements.
Most websites nowadays are structured using 'div' elements. These are highly customisable, both in look and interactivity.
Let's have another look at the heinously-designed page from the 'GeoCities Era' section of this website. What happens if tables are replaced with 'div' elements?
Here is a title.
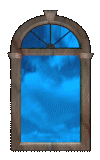
This paragraph of text has a window either side of it.
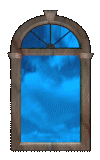

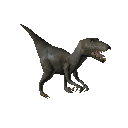
Fill this part with text. It stretches over 2 columns. Table cells allow for rigid positioning of objects. There is a T-Rex to the left of this paragraph.
<!DOCTYPE ... >
<html xmlns ... >
<head>
<title>Title goes here.</title>
<style type="text/css">
#geo_contentttable {
position: relative;
display: table;
border-spacing: 0px;
width: 100%;
}
.geo_content {
display: inline-block;
max-width: 350px;
text-align: center;
font-family: 'Century Gothic';
color: #f00;
}
.geo_divider{
width: 100%;
text-align: center;
}
#geo_content2{
position: relative;
display: table;
border-spacing: 1px;
width: 100%;
background-color: #ff3;
color: #00f;
}
.geo_content2section{
display: inline-block;
max-width: 430px;
font-family: 'Verdana';
text-align: right;
vertical-align: top;
}
</style>
</head>
<body>
<div id="geocities-preview">
<div id="geo_contenttable">
<div class="marquee"><h1>Here is a title.</h1></div>
<div class="geo_content">
<img src="window.gif" alt="window" />
</div>
<div class="geo_content">
<p>This paragraph of text has a window either side of it.</p>
</div>
<div class="geo_content">
<img src="window.gif" alt="window" />
</div>
</div>
<div class="geo_divider">
<img src="animateline.gif" alt="divider" />
</div>
<div id="geo_content2">
<div class="geo_content2section">
<img src="rex.gif" alt="T-Rex" />
</div>
<div class="geo_content2section">
<p>Fill this part with text. It stretches over 2 columns. Table cells allow for rigid positioning of objects. There is a T-Rex to the left of this paragraph.</p>
</div>
</div>
</div>
</body>
</html>
The live demo looks the same, but the table and the cells within it have been replaced by 'div' elements. Each of the 'div' elements need a style associated with them. One other thing that changed was the 'marquee' HTML element - it has been removed, and replaced with a 'div' element under the class 'marquee'.
Marquee as an HTML element is deprecated (no longer used), which is probably for the best, but it is possible to achieve this effect using CSS. An example of this is shown below. CSS3 has the ability to handle a wide variety of different animations, and that is without even considering JavaScript.
.marquee {
height: 50px;
overflow: hidden;
position: relative;
background: #0f0;
}
.marquee [tag to target] {
position: absolute;
width: 100%;
height: 100%;
margin: 0;
line-height: 50px;
text-align: left;
-moz-animation: marquee [time in s] linear infinite alternate;
-webkit-animation: marquee [time in s] linear infinite alternate;
animation: marquee [time in s] linear infinite alternate;
}
@-moz-keyframes marquee {
0% { -moz-transform: translateX(65%); }
100% { -moz-transform: translateX(0%); }
}
@-webkit-keyframes marquee {
0% { -webkit-transform: translateX(65%); }
100% { -webkit-transform: translateX(0%); }
}
We're still using the concept of separating the style (the CSS) from the information (the HTML), but as you can see, if there were more elements on the page the 'view code' section will end up being monolithic and difficult to follow.
It turns out that our concerns can be separated one step further - by keeping the HTML, CSS, and JavaScript (JS) in separate files. We will cover this in the 'Separation of Concerns' section.